Hiring Workers and Monitoring Job Progress (Jobs)
Jobs represent an individual Wonoloer (Worker) performing a Job Request. The number of Jobs associated with a Job Request correspond to the slots defined on the Job Request. Jobs allow Requesters to track and manage the status of the Wonoloer as they perform their assigned task.
Onboarding Workers
Workers are “hired” by posting to the Assign a Job endpoint of the Jobs service, using the worker’s User id and the Job Request id.
curl --request POST \
--url https://test.wonolo.com/api_v2/jobs/assign?token=QwErTyAsDfZxC \
--header 'content-type: application/json' \
--data '{"job_request_id":1234,"worker_id":5678,"requestor_notes":"Solid hire!"}'
var data = JSON.stringify({
"job_request_id": 1234,
"worker_id": 5678,
"requestor_notes": "Solid hire!"
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function () {
if (this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://test.wonolo.com/api_v2/jobs/assign?token=QwErTyAsDfZxC");
xhr.setRequestHeader("Content-Type", "application/json");
xhr.setRequestHeader("Accept", "*/*");
xhr.setRequestHeader("Cache-Control", "no-cache");
xhr.setRequestHeader("Host", "test.wonolo.com");
xhr.setRequestHeader("Accept-Encoding", "gzip, deflate");
xhr.setRequestHeader("Content-Length", "72");
xhr.setRequestHeader("Connection", "keep-alive");
xhr.setRequestHeader("cache-control", "no-cache");
xhr.send(data);
Job States
As with Job Requests, one of the most important attributes of the Job entity is its state
, which can be used to monitor the progress of the job, based on how the worker and employer are interacting with the job.
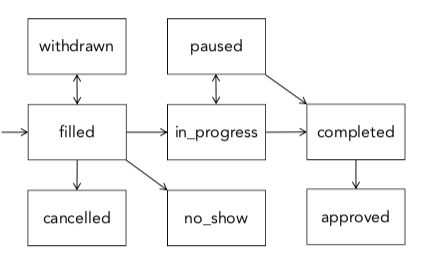
The following job states exist:
filled | The Worker has accepted a Job but has not started it yet – i.e. they pressed “Accept” in the app. |
withdrawn | The Worker withdrew from the Job (NB: if the Worker accepts the Job again, the same Job will return to the filled state) |
cancelled | The Job has been cancelled by the Requestor |
in_progress | The Worker has pressed “Start” in the Wonolo app |
paused | The Worker has paused the job to take a break |
completed | The Worker has pressed “Complete” in the Wonolo app |
approved | The Requestor has approved the job as done |
no_show | The Requestor has marked that the Worker did not show up for the Job |
Time and Attendance
Each Job includes an array of “transitions” which records the date and time of each change in the Job’s state. These transitions can be used to monitor a worker’s status with a transition to in_progress
equating to “clocking in” and a transition to paused
or completed
equating to “clocking out.”
Below is an example of transitions in a typical Jobs object.
"transitions": [
{
"id": 5644,
"job_id": 2381,
"created_at": "2017-07-14T19:49:41Z",
"name": "approved",
"latitude": null,
"longitude": null
},
{
"id": 5629,
"job_id": 2381,
"created_at": "2017-07-14T19:30:41Z",
"name": "completed",
"latitude": "34.02566650642284",
"longitude": "-81.0921330185882"
},
{
"id": 5628,
"job_id": 2381,
"created_at": "2017-07-14T19:30:38Z",
"name": "in_progress",
"latitude": "34.02566650642284",
"longitude": "-81.0921330185882"
},
{
"id": 5534,
"job_id": 2381,
"created_at": "2017-06-15T14:55:23Z",
"name": "filled",
"latitude": null,
"longitude": null
},
{
"id": 5527,
"job_id": 2381,
"created_at": "2017-06-15T14:54:35Z",
"name": "filled_flexible",
"latitude": null,
"longitude": null
}
]
Ending Jobs
Jobs may be cancelled by posting a PATCH request to the Jobs service with a reason for the cancellation as the cancelled_reason
property.
Webhooks for Jobs
You may monitor the state of Jobs by receiving callbacks to your application via Webhooks.
See this section for information on how to configure you application to use the Wonolo API Webhooks.
Below is an example of the JSON payload of a job state change call back.
{
"id": 90909090,
"created_at": "2019-11-07T21:27:59.423-08:00",
"event": "job.state_change",
"resource": {
"id": 234567,
"state": "completed",
"job_request_id": 89898989,
"created_at": "2019-10-31T11:55:04.085-07:00",
"updated_at": "2019-11-07T21:27:59.224-08:00",
"confirmed_at": "2019-10-31T13:31:17.687-07:00",
"started_at": "2019-11-07T16:25:23.711-08:00",
"completed_at": "2019-11-07T21:27:59.222-08:00",
"hours_worked": 0,
"actual_duration": 18155,
"finished_at": null,
"time_to_start": 618846,
"adjusted_wage": null,
"requestor_notes": null,
"classification": "1099",
"w2_hourly_rate": "",
"w2_payment_ref": null,
"w2_pay_status": null,
"worker": {
"id": 234567,
"external_id": null,
"type": "Worker",
"first_name": "Jane",
"last_name": "Doe",
"avatar_url": "https://avataraservername.exmpl/uploads/avatar1234.png",
"city": "San Francisco",
"zip": "94115",
"rating": "5.0",
"suspended": false,
"suspended_until": null,
"latitude": "37.7952",
"longitude": "-122.4028",
"workplace_image_url": null,
"logo_url": null,
"business_name": null,
"email": "[email protected]",
"phone": "+15555555555",
"gender": null,
"w2_employee_id": null,
"w2_onboarding_status": null,
"w2_onboarding_started": null,
"dob": null,
"ssn": "",
"address": null,
"address_state": null,
"drug_tested": null,
"updated_at": "2019-11-07T21:27:59.401-08:00",
"w2_address_state": null,
"w2_onboarding_completed": null,
"lower_pool": null,
"lower_pool_until": null,
"home_latitude": "37.7952",
"home_longitude": "-122.4028",
"referred_by": null,
"tracking_code": null,
"onboarding_appt_time": null,
"user_badges": [
{
"id": 219523,
"user_id": 234567,
"badge_id": 1834,
"expires_at": null,
"created_at": "2019-10-24T02:19:56.885-07:00",
"suspended": false,
"category": "",
"updated_at": "2019-10-24T02:19:56.885-07:00",
"discount_code": null
},
{
"id": 219524,
"user_id": 234567,
"badge_id": 4702,
"expires_at": null,
"created_at": "2019-10-24T02:19:56.896-07:00",
"suspended": false,
"category": "",
"updated_at": "2019-10-24T02:19:56.896-07:00",
"discount_code": null
},
{
"id": 219525,
"user_id": 234567,
"badge_id": 5192,
"expires_at": null,
"created_at": "2019-10-24T02:19:56.907-07:00",
"suspended": false,
"category": "",
"updated_at": "2019-10-24T02:19:56.907-07:00",
"discount_code": null
},
{
"id": 219526,
"user_id": 234567,
"badge_id": 5193,
"expires_at": null,
"created_at": "2019-10-24T02:19:56.917-07:00",
"suspended": false,
"category": "",
"updated_at": "2019-10-24T02:19:56.917-07:00",
"discount_code": null
},
{
"id": 219522,
"user_id": 234567,
"badge_id": 5863,
"expires_at": null,
"created_at": "2019-10-24T02:19:56.872-07:00",
"suspended": false,
"category": "",
"updated_at": "2019-10-24T02:19:56.872-07:00",
"discount_code": null
}
]
},
"employer": {
"id": 1234,
"type": "Employer",
"first_name": "Training",
"last_name": "Incorporated",
"avatar_url": null,
"city": null,
"zip": null,
"rating": null,
"suspended": false,
"suspended_until": null,
"logo_url": null,
"business_name": "Wonolo Training In",
"email": "[email protected]",
"phone": "",
"address": null,
"address_state": null,
"title": "",
"role": "requestor",
"customer_id": 12345,
"updated_at": "2019-05-08T09:11:38.940-07:00"
},
"transitions": [
{
"id": 8809925,
"job_id": 234567,
"created_at": "2019-11-08T05:27:59Z",
"name": "completed",
"latitude": "42.3530213",
"longitude": "-73.7473045"
},
{
"id": 8805816,
"job_id": 234567,
"created_at": "2019-11-08T00:25:23Z",
"name": "in_progress",
"latitude": "37.7952",
"longitude": "-122.4028"
},
{
"id": 8666297,
"job_id": 234567,
"created_at": "2019-10-31T20:31:17Z",
"name": "filled",
"latitude": null,
"longitude": null
},
{
"id": 8663727,
"job_id": 234567,
"created_at": "2019-10-31T18:55:04Z",
"name": "pending",
"latitude": null,
"longitude": null
}
]
}
}
Updated about 5 years ago